SDK reference
Overview
Embedded Checkout adds a global Checkout
object, with a single initiate
method.
type CheckoutOptions = {
/**
* Checkout id retrieved from an API call to /v2/checkout
*/
checkoutId: string;
/**
* Entity id, needs to match the entity id used to create the checkout.
*/
key: string;
/**
* Options for the Checkout UI.
*/
options?: {
/**
* Theming options.
*
* Will also apply to selected payment methods.
*/
theme?: {
brand?: {
primary?: string;
secondary?: string;
};
cards?: {
background?: string;
backgroundHover?: string;
};
};
/**
* Custom ordering of payment methods.
*
* e.g.
* {
* card: 1,
* mobicred: 2,
* eftsecure: 3,
* }
*/
ordering?: Record<string, number>;
};
/**
* Event handlers.
*
* Hook into Checkout events, and perform actions when they occur.
*/
events?: {
/**
* Event fired when Checkout has completed successfully.
* @param event Details about the transaction.
* @returns Void
*/
onCompleted?: (event: CompletedEvent) => void;
/**
* Event fired when Checkout was cancelled by the user.
* @param event Details about the Checkout.
* @returns Void
*/
onCancelled?: (event: CheckoutEvent) => void;
/**
* Event fired when Checkout has timed out.
* @param event Details about the Checkout.
* @returns Void
*/
onExpired?: (event: CheckoutEvent) => void;
};
}
type RenderCheckout = {
/**
* Render Embedded Checkout into an HTML element.
* @param container ID of the HTML element to render Embedded Checkout into.
*/
render: (container: string) => void;
/**
* Remove Embedded Checkout from being rendered.
*
* NOTE: The same Checkout ID cannot be reused for another render attempt, a new ID will need to be generated.
*/
unmount: () => void;
}
type Checkout = {
/**
* Initiate a Checkout UI experience.
* @param options Options for the Checkout experience.
* @returns An object that can be used to render the Checkout UI or to unmount the Checkout UI.
*/
initiate: (options: CheckoutOptions) => RenderCheckout;
};
Events
Embedded Checkout supports events. When you specify a handler for an event, Embedded Checkout calls that event and does not redirect. If you don't specify a handler, Embedded Checkout redirects.
Event | Description |
---|---|
onCompleted | The customer completes a payment and the checkout experience. |
onCancelled | The customer cancels the checkout experience. |
onExpired | The checkout experience expires (customers have 30 minutes to complete a payment). |
{
amount: number;
checkoutId: string;
currency: string;
merchantTransactionId: string;
paymentType: "DB";
result: { code: string; description: string };
signature: string;
timestamp: Date;
id: string;
merchant: {
name: string;
};
paymentBrand: string;
resultDetails: {
AcquirerResponse: string;
ConnectorTxID1: string;
ExtendedDescription: string;
};
}
{
amount: number;
checkoutId: string;
currency: string;
merchantTransactionId: string;
paymentType: "DB";
result: { code: string; description: string };
signature: string;
timestamp: Date;
id: string;
}
{
amount: number;
checkoutId: string;
currency: string;
merchantTransactionId: string;
paymentType: "DB";
result: { code: string; description: string };
signature: string;
timestamp: Date;
id: string;
}
Theming
Embedded Checkout enables you to set the following colours:
-
Brand primary: Controls the cancel button font and border hover colour, the currency font colour, and the payment method card border hover colour.
Embedded Checkout with primary colour set to orange.
-
Brand secondary: Not used.
-
Cards background: Controls the payment method card background colour.
Embedded Checkout with payment method card background colour set to blue.
-
Cards background hover: Controls the payment method card background hover colour.
Embedded Checkout with payment method card background hover colour set to green.
Set the primary, secondary, and background colours as follows, ensuring that you use hexadecimal colour codes, for example, #000000
for black.
{
brand: {
primary: string;
secondary: string;
};
cards: {
background: string;
backgroundHover: string;
};
}
Customise payment method order
Embedded Checkout enables you to specify the order of payment methods. This works by specifying an object with the payment method name and a number; lower numbers mean higher priority.
See the Payment methods section for more information on payment method parameter names.
{
MASTERPASS: 1,
"1FORYOU": 2,
MOBICRED: 3,
CARD: 4,
}
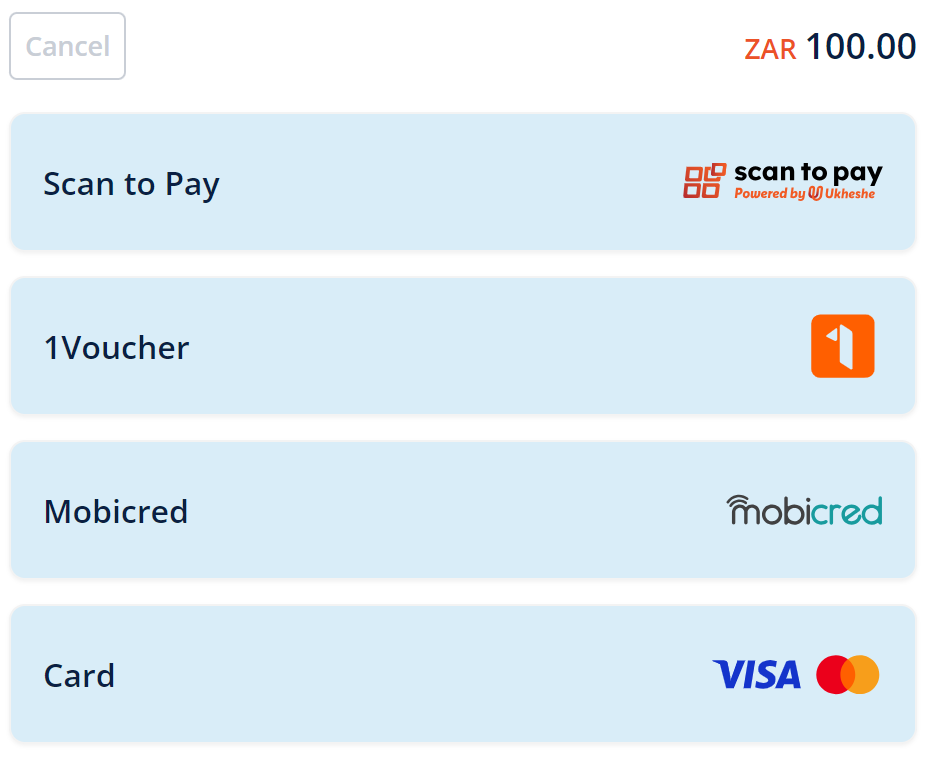
Example Embedded Checkout with custom colours and payment method order; note that not all payment methods are available in all regions or for all currencies.
Updated 3 months ago