Payment Links authentication
Overview
To use the Payment Links API, merchants must retrieve their API credentials from the Dashboard as described on Payment Links on the Dashboard.
Before making calls to the Payment Links API, merchants must get an access token from the Authentication API.
The Authentication API endpoints are:
- Live:
https://dashboard.peachpayments.com
- Sandbox:
https://sandbox-dashboard.peachpayments.com
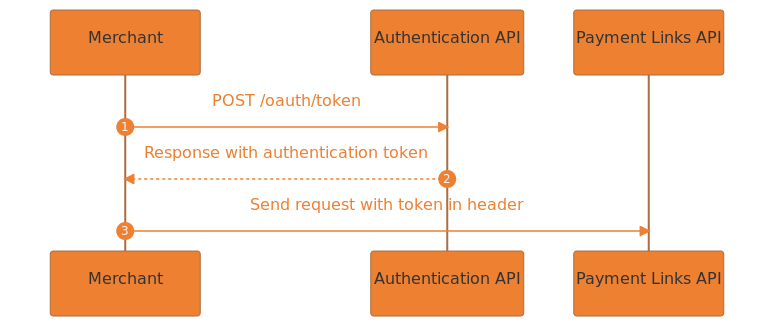
Authentication flow.
-
Make a POST
{peach-auth-service}/api/oauth/token
request to the Authentication API with your client ID, client secret, and merchant ID to generate an access token.curl --location --request POST '{peach-auth-service}/api/oauth/token' --header 'content-type: application/json' --data-raw '{ "clientId": "{{clientId}}", "clientSecret": "{{clientSecret}}", "merchantId": "{{merchantId}}" }'
-
The Authentication API responds with an access token.
{ "access_token": "<access token>", "expires_in": "<token expiry duration in seconds>", "token_type": "Bearer" }
-
Make calls to the Payment Links API with the access token in the authorisation header, for example,
Authorization: Bearer {access_token}
.
- You can reuse the access token from step 2 for multiple API calls.
- When the token expires, you need to generate a new one.
See the Postman collection for more details on API authentication.
Code snippet
async function authenticate(clientId, clientSecret, merchantId) {
let response = await fetch("<peach auth service>/api/oauth/token", {
method: "POST",
body: JSON.stringify({
clientId,
clientSecret,
merchantId
})
});
if (response.ok) {
let body = await response.json();
return body.access_token;
} else {
throw new Error("Unable to authenticate");
}
}
async function getPaymentLinkDetails(token, paymentLinkId) {
let response = await fetch("<payment links api>/api/payments/" + paymentLinkId, {
headers: new Headers({
"Authorization": "bearer " + token
})
});
if (response.ok) {
return await response.json();
} else {
throw new Error("Unable to retrieve Payment Link details");
}
}
let bearerToken = await authenticate(clientId, clientSecret, merchantId);
let paymentLink = await getPaymentLinkDetails(bearerToken, paymentLinkId);
Updated 6 months ago